This is my own compilation of some of the interesting C programs. Welcome to C Questions blog. Welcome you to publish your own such interesting C puzzles. And, well recently I have added some C++ stuff as well, hope you will enjoy them. Thanks
Friday, December 19, 2008
About printf Part 2
1.
main() {
const int i=4;
float j;
j = ++i;
printf("%d %f", i,++j);
}
Answer:
Compiler error
Explanation:
i is a constant. You cannot change the value of constant.
2.
int i=10;
main() {
extern int i; {
int i=20;{
const volatile unsigned i=30;
printf("%d",i);
}
printf("%d",i);
}
printf("%d",i);
}
Answer:
30,20,10
Explanation:
'{' introduces new block and thus new scope. In the innermost block i is declared as, const volatile unsigned which is a valid declaration. i is assumed of type int. So printf prints 30. In the next block, i has value 20 and so printf prints 20. In the outermost block, i is declared as extern, so no storage space is allocated for it. After compilation is over the linker resolves it to global variable i (since it is the only variable visible there). So it prints i's value as 10.
3.
aaa() {
printf("hi");
}
bbb(){
printf("hello");
}
ccc(){
printf("bye");
}
main(){
int (*ptr[3])();
ptr[0]=aaa;
ptr[1]=bbb;
ptr[2]=ccc;
ptr[2]();
}
Answer:
bye
Explanation:
ptr is array of pointers to functions of return type int.ptr[0] is assigned to address of the function aaa. Similarly ptr[1] and ptr[2] for bbb and ccc respectively. ptr[2]() is in effect of writing ccc(), since ptr[2] points to ccc.
4.
main(){
FILE *ptr;
char i;
ptr=fopen("zzz.c","r");
while((i=fgetc(ptr))!=EOF)
printf("%c",i);
}
Answer:
Contents of zzz.c.
5.
void main(){
void *v;
int integer=2;
int *i=&integer;
v=i;
printf("%d",(int*)*v);
}
Answer:
Compiler Error. We cannot apply indirection on type void*.
Explanation:
Void pointer is a generic pointer type. No pointer arithmetic can be done on it. Void pointers are normally used for,
1>
Passing generic pointers to functions and returning such pointers.
2>
As a intermediate pointer type.
3>
Used when the exact pointer type will be known at a later point of time.
6.
void main(){
while(1){
if(printf("%d",printf("%d")))
break;
else
continue;
}
}
Answer:
Garbage values.
Explanation:
The inner printf executes first to print some garbage value. The printf returns no of characters printed and this value also cannot be predicted. Still the outer printf prints something and so returns a non-zero value. So it encounters the break statement and comes out of the while statement.
7.
main(){
unsigned int i=10;
while(i-->=0)
printf("%u ",i);
}
Answer:
9 8 7 6 5 4 3 2 1 0 65535 65534…..
Explanation:
Since i is an unsigned integer it can never become negative. So the expression i-- >=0 will always be true, leading to an infinite loop.
8.
main(){
int a[10];
printf("%d",*a+1-*a+3);
}
Answer:
4
Explanation:
*a and -*a cancels out. The result is as simple as 1 + 3 = 4 !
9.
main(){
unsigned int i=65000;
while(i++!=0);
printf("%d",i);
}
Answer:
1
Explanation:
Note the semicolon after the while statement. When the value of i becomes 0 it comes out of while loop. Due to post-increment on i the value of i while printing is 1.
10.
void pascal f(int i,int j,int k){
printf(“%d %d %d”,i, j, k);
}
void cdecl f(int i,int j,int k){
printf(“%d %d %d”,i, j, k);
}
main(){
int i=10;
f(i++,i++,i++);
printf(" %d\n",i);
i=10;
f(i++,i++,i++);
printf(" %d",i);
}
Answer:
10 11 12 13
12 11 10 13
Explanation:
Pascal argument passing mechanism forces the arguments to be called from left to right. cdecl is the normal C argument passing mechanism where the arguments are passed from right to left.
11.
What is the output of the program given below:
main(){
signed char i=0;
for(;i>=0;i++) ;
printf("%d\n",i);
}
Answer:
-128
Explanation:
Notice the semicolon at the end of the for loop. THe initial value of the i is set to 0. The inner loop executes to increment the value from 0 to 127 (the positive range of char) and then it rotates to the negative value of -128. The condition in the for loop fails and so comes out of the for loop. It prints the current value of i that is -128.
12.
main(){
unsigned char i=0;
for(;i>=0;i++) ;
printf("%d\n",i);
}
Answer:
infinite loop.
Explanation:
The difference between the previous question and this one is that the char is declared to be unsigned. So the i++ can never yield negative value and i>=0 never becomes false so that it can come out of the for loop.
13.
main(){
char i=0;
for(;i>=0;i++) ;
printf("%d\n",i);
}
Answer:
Behavior is implementation dependent.
Explanation:
The detail if the char is signed/unsigned by default is implementation dependent. If the implementation treats the char to be signed by default the program will print –128 and terminate. On the other hand if it considers char to be unsigned by default, it goes to infinite loop.
Rule:
You can write programs that have implementation dependent behavior. But dont write programs that depend on such behavior.
That's it for today...
Thanks for reading.
About printf Part 1
1.
void main(){
int const * p=5;
printf("%d",++(*p));
}
Answer:
Compiler error: Cannot modify a constant value.
Explanation:
p is a pointer to a "constant integer". But we tried to change the value of the "constant integer".
2.
main(){
float me = 1.1;
double you = 1.1;
if(me==you)
printf("I love U");
else
printf("I hate U");
}
Answer:
I hate U
Explanation:
For floating point numbers (float, double, long double) the values cannot be predicted exactly. Depending on the number of bytes, the precision with of the value represented varies. Float takes 4 bytes and long double takes 10 bytes. So float stores 0.9 with less precision than long double.
Rule of Thumb:
Never compare or at-least be cautious when using floating point numbers with relational operators (== , >, <, <=, >=,!= ) .
3.
main(){
extern int i;
i=20;
printf("%d",i);
}
Answer:
Linker Error : Undefined symbol '_i'
Explanation:
extern storage class in the following declaration,
extern int i;
specifies to the compiler that the memory for i is allocated in some other program and that address will be given to the current program at the time of linking. But linker finds that no other variable of name i is available in any other program with memory space allocated for it. Hence a linker error has occurred .
4.
main(){
char *p;
printf("%d %d ",sizeof(*p),sizeof(p));
}
Answer:
1 2
Explanation:
The sizeof() operator gives the number of bytes taken by its operand. P is a character pointer, which needs one byte for storing its value (a character). Hence sizeof(*p) gives a value of 1. Since it needs two bytes to store the address of the character pointer sizeof(p) gives 2.
5.
main(){
int i=3;
switch(i) {
default:printf("zero");
case 1: printf("one");
break;
case 2:printf("two");
break;
case 3: printf("three");
break;
}
}
Answer :
three
Explanation :
The default case can be placed anywhere inside the loop. It is executed only when all other cases doesn't match.
6.
main(){
char string[]="Hello World";
display(string);
}
void display(char *string){
printf("%s",string);
}
Answer:
Compiler Error : Type mismatch in redeclaration of function display
Explanation :
In third line, when the function display is encountered, the compiler doesn't know anything about the function display. It assumes the arguments and return types to be integers, (which is the default type). When it sees the actual function display, the arguments and type contradicts with what it has assumed previously. Hence a compile time error occurs.
7.
main(){
int i;
printf("%d",scanf("%d",&i)); // value 10 is given as input here
}
Answer:
1
Explanation:
Scanf returns number of items successfully read.Here 10 is given as input which should have been scanned successfully. So number of items read is 1.
8.
main(){
extern int i;
i=20;
printf("%d",sizeof(i));
}
Answer:
Linker error: undefined symbol '_i'.
Explanation:
extern declaration specifies that the variable i is defined somewhere else. The compiler passes the external variable to be resolved by the linker. So compiler doesn't find an error. During linking the linker searches for the definition of i. Since it is not found the linker flags an error.
9.
main(){
printf("%d", out);
}
int out=100;
Answer:
Compiler error: undefined symbol out in function main.
Explanation:
The rule is that a variable is available for use from the point of declaration. Even though a is a global variable, it is not available for main. Hence an error.
10.
main(){
extern out;
printf("%d", out);
}
int out=100;
Answer:
100
Explanation:
This is the correct way of writing the previous program.
11.
int i,j;
for(i=0;i<=10;i++){ j+=5; assert(i<5); } Answer: Runtime error: Abnormal program termination. assert failed (i<5),
Explanation:
asserts are used during debugging to make sure that certain conditions are satisfied. If assertion fails, the program will terminate reporting the same. After debugging use,
#undef NDEBUG
and this will disable all the assertions from the source code. Assertion is a good debugging tool to make use of.
12.
main(){
char name[10],s[12];
scanf(" \"%[^\"]\"",s);
}
How scanf will execute?
Answer:
First it checks for the leading white space and discards it.Then it matches with a quotation mark and then it reads all character upto another quotation mark.
13.
What is the problem with the following code segment?
while ((fgets(receiving array,50,file_ptr)) != EOF) ;
Answer & Explanation:
fgets returns a pointer. So the correct end of file check is checking for != NULL.
That's it.
Monday, August 18, 2008
Windows Debugging
Windows Debugging
I rarely have to debug things under windows (thankfully) but that means when I do I have forgotten all the knowledge that I had learnt so slowly the time before. Hopefully this will remind me of things and help others as well.
* Process Monitor (Microsoft/SysInternals) can do the equivalent of truss. Use a filter to match your process.
* Process Explorer (Microsoft/SysInternals) can do the equivalent of lsof.
There are a couple of other useful free tools from Microsoft/SysInternals http://www.sysinternals.com
* Depends - similar to ldd to help with Windows DLL hell.
* Pstools - provides a set of command line Unix like process utils, such as pskill, psinfo.
A useful stand alone debugger is available from Microsoft http://www.microsoft.com/whdc/devtools/debugging plus other debugging resources.
At times when you need to kill a process we use "kill
taskkill /F /IM
In fact taskkill has got several other options also. You can list all the options by "taskkill /?" command.
"ps" command is used to list the running processes in Unix. We use "tasklist" in Windows.
REMEMBER: Windows is case insensitive, unlike Unix.
If you are working with Windows Event Logs then "EventCreate" is the command to generate a Windows Event Log entry. A sample is given below:
eventcreate /L Application /T ERROR /SO GMI0 /ID 1 /D "Test message %DATE% %TIME%"
Here is a simple script in Unix, which will actually create a batch file for Windows Environment which creates large number of events in Windows Event Log.
#! /bin/ksh
export COUNTER SOURCE
SOURCE=0
while [ $SOURCE -le 100 ]
do
SOURCE=`expr $SOURCE + 1`
COUNTER=0
while [ $COUNTER -le 1000 ]
do
COUNTER=`expr $COUNTER + 1`
echo "eventcreate /L Application /T ERROR /SO TESTAPP${SOURCE} /ID $COUNTER /D \"TESTAPP${SOURCE} : $COUNTER : Test event message : %DATE% %TIME%\""
done
done
-- Thanks to Jim Hutchinson for this script.
Wednesday, July 23, 2008
Compiling the latest 2.6 Linux Kernel
Let's compile the latest 2.6 Linux kernel.
Here are the steps:
1. Get the latest kernel from the www.kernel.org
2. Untar the kernel into /usr/src/
tar xvfj linux2.6.26.tar.bz2 -C /usr/src/
This will create a directory /usr/src/linux2.6.26/ and untar the linux kernel into this directory.
3. Goto the kernel $TOPDIR
$cd /usr/src/linux2.6.26/
4. make menuconfig
Here are the steps:
1. Get the latest kernel from the www.kernel.org
2. Untar the kernel into /usr/src/
tar xvfj linux2.6.26.tar.bz2 -C /usr/src/
This will create a directory /usr/src/linux2.6.26/ and untar the linux kernel into this directory.
3. Goto the kernel $TOPDIR
$cd /usr/src/linux2.6.26/
4. make menuconfig
Friday, May 23, 2008
Linux Questions

Here I have collected some of my linux experiences bundled in this blog. Hope you guys will enjoy this blog we well like the earlier ones. Thanks a lot, and keep reading. Here it is:
Q1. How to get the version of Linux installed in the system?
Ans:
cat /etc/redhat-release
Q2. How to get the version of Linux Kernel?
Ans:
uname -a
Q3. How to check the current runlevel?
Ans:
$runlevel
Red Hat Linux and Fedora
Red Hat as well as most of its derivatives uses runlevels like this:
- 0 - Halt
- 1 - Single user
- 2 - Not used/User definable
- 3 - Full multi-user, console logins only
- 4 - Not used/User definable
- 5 - Full multi-user, with display manager as well as console logins
- 6 - Reboot
Ref: http://en.wikipedia.org/wiki/Runlevel.
Q4.
Ans:
Q5. How to start the computer in single user mode?
Ans:
a) Type 'single' at the end of the boot line
b) Type 'init 1' at the end of the boot line
Q6. How to boot linux in super user mode?
Ans:
Change the boot loader kernel booting option while starting the kernel just after the boot loader starts.
"init 1" should be passed as the last parameter, and then b to boot with this parameter. Linux will boot in single user mode. In case you want to change the root passwd, this is the time, use the passwd command to change the root passwd.
Q7. What is the equivalent command in HPUX for 'ipconfig -a'?
Ans:
There is no equivalent command of 'ipconfig -a' in HPUX, rather use the following script code in command line:
for lan in $(lanscan | grep lan | awk '{print $5}') ; do
echo $lan
ifconfig $lan
done
Q8.How to configure different services in start-up?
Ans:
'chkconfig' is the command.
Graphically, Red Hat / Fedora Core GUI: system-config-services (and redhat-config-services)
Red Hat/Fedora Core text console services selection tool: /usr/sbin/ntsysv
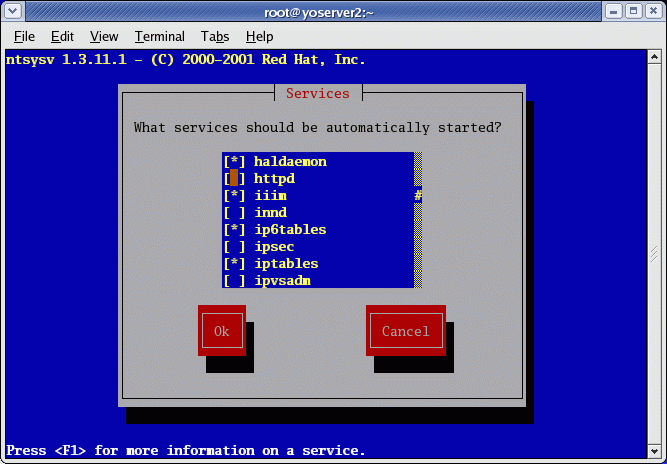
Q9.What Is a core File?
Ans: A core file is created when a program terminates unexpectedly, due to a bug, or a violation of the operating system's or hardware's protection mechanisms. The operating system kills the program and creates a core file that programmers can use to figure out what went wrong. It contains a detailed description of the state that the program was in when it died.
If would like to determine what program a core file came from, use the file command, like this:
$ file core
That will tell you the name of the program that produced the core dump. You may want to write the maintainer(s) of the program, telling them that their program dumped core.
Q10. How To Enable or Disable Core Dumps
Ans: By using the ulimit command in bash, the limit command in tcsh, or the rlimit command in ksh. See the appropriate manual page for details.
This setting affects all programs run from the shell (directly or indirectly), not the whole system.
If you wish to enable or disable core dumping for all processes by default, you can change the default setting in linux/sched.h. Refer to definition of INIT_TASK, and look also in linux/resource.h.
PAM support optimizes the system's environment, including the amount of memory a user is allowed. In some distributions this parameter is configurable in the /etc/security/limits.conf file.
Q11. What are different types of open source licenses?
Ans:
Open source licenses may be broadly categorized into the following types: (1) those that apply no restrictions on the distribution of derivative works (we will call these Non-Protective Licenses because they do not protect the code from being used in non-Open Source applications); and (2) those that do apply such restrictions (we will call these Protective Licenses because they ensure that the code will always remain open/free).
To better appreciate the nature of these licenses, it is helpful to picture software licenses on a continuum based on the rights in copyright extended to the licensee.
Software that has been placed in the public domain is free of all restrictions, all rights under copyright having been granted to the public at large. Licensors of Non-Protective Open Source licenses retain their copyright, but they grant all rights under copyright to the licensee. Licensors of Protective Open Source licenses retain their copyright, grant all rights under copyright to the licensee, but apply at least one restriction, typically that the redistribution of the software, whether modified or unmodified, must be under the same license. Licensors of propriety licenses retain their copyright and only grant a few rights under copyright, typically only the rights to perform and display. The BSD license is an example of a Non-Protective Open Source license and the GNU General Public License is an example of a Protective Open Source license.
List of some open source licensed products::
Non-Protective Open Source licenses include: Academic Free License v.1.2; Apache Software License v.1.1; Artistic; Attribution Assurance license; BSD License; Eiffel Forum License; Intel Open Source License for CDSA/CSSM Implementation; MIT License; Open Group Test Suite License; Q Public License v.1.0; Sleepycat License; Sun Industry Standards Source License; University of Illinois/NCSA Open Source License; Vovida Software License v.1.0; W3C Software Notice and License; X.Net, Inc. License; zlib/libpng License; and Zope Public License v.2.0.
Protective Open Source licenses include: Apple Public Source License v.1.2; Artistic License; Common Public License v.1.0; GNU General Public License v.2.0; GNU Lesser General Public License v.2.1; IBM Public License v.1.0; Jabber Open Source License v.1.0; MITRE Collaborative Virtual Workspace License; Motosoto Open Source License v.0.9.1; Mozilla Public License v.1.0 and v.1.1; Nethack General Public License; Noika Open Source License v.1.0a; OCLC Research Public License v.1.0; Open Software License v.1.1; Python License; Python Software Foundation License v.2.1.1; Ricoh Source Code Public License v.1.0; and Sun Public License v.1.0.
Q12:
Here is a nice link which talks about the Command-line Calculator in Linux
Ans:
http://linux.byexamples.com/archives/42/command-line-calculator-bc/
Q13: What is copyleft?
Ans:
Copyleft is a great license originally designed for software which can be applied to images, text, pictures and any other work for that matter. The license says the work is free and must remain free. You can do what you want with the work as long as it remains in the commons. You can not take a Copyleft picture and put it on a website that has a copyright notice restricting others from freely using the picture, but you can use it if you are using Copyleft yourself.
Copyleft is a very good idea for music also. Most so-called "samples" used to create modern music are owned by large corporations who insist you buy their music using a polluting, non-sustainable media called DVD discs. Copyleft music can be distributed freely, meaning people will promote your bands music to the millions at no cost to you.
Monday, March 03, 2008
SIGBUS Vs SIGSEGV
Here is a special blog, this one talks about the subtle differences between two of Linux Signals: SIGBUS and SIGSEGV.
There are different reasons behind the generation of SIGBUS or SIGSEGV. And here we analysis the difference between them, how a SIGBUS error generates and how it is different from SIGSEGV which is more obviously occurs.
The reason of SIGSEGV and SIGBUS are:
There are different reasons behind the generation of SIGBUS or SIGSEGV. And here we analysis the difference between them, how a SIGBUS error generates and how it is different from SIGSEGV which is more obviously occurs.
The reason of SIGSEGV and SIGBUS are:
Signal Code Reason SIGSEGV SEGV_MAPERR address not mapped to object SEGV_ACCERR invalid permissions for mapped object ____________________________________________________________ SIGBUS BUS_ADRALN invalid address alignment BUS_ADRERR non-existent physical address BUS_OBJERR object specific hardware error SIGBUS: signal number=10; SIGSEGV: signal number=11; Here is an sample program which will generate a SIGBUS error: //file:sigbus.cint main(int argc, char **argv) { int testvar = 0x12345678; int *testvarp; testvarp = &testvar; printf("testvarp was %lx\n", testvarp); printf("testvar was %lx\n", *testvarp); testvarp = (int *)(((char *)testvarp) + 1); printf("testvarp is %lx\n", testvarp); printf("testvar is %lx\n", *testvarp);
}
$gcc sigbus.c devtest6:/home/jkongkon/sea>./a.out testvarp was ffbef224 testvar was 12345678 testvarp is ffbef225 Bus Error
devtest6:/home/jkongkon/sea>uname -a SunOS devtest6 5.8 Generic_117350-39 sun4u sparc SUNW,Ultra-80 devtest6:/home/jkongkon/sea> The above sample code illustrate how a SIGBUS got generated as a result of a "invalid address alignment", with the error code BUS_ADRALN. This example illustrates that a SIGBUS can generate as the result of a programming error. Another reason where SIGBUS can generate is explained below: You are currently using a external I/O device by mapping the device memory mapping into the system memory (Memory mapped I/O). You have used it. And now, you have disconnected it gracefully. But, somehow your code is trying to use an previouslt used address still in your code. The result in this case will be an SIGBUS, the reason is BUS_ADRERR, "non-existent physical address". I guess, everyone who does some system programming has got SIGSEGV at some point. The simplest way to get a SIGSEGV is this: main(){ int *p=(int*)0; printf("*p=%d\n", *p); } Result="Segmentation Fault". Here are some reasons why we get these signals: SIGSEGV <-- Attempted write into a region mapped as read-only. SIGBUS <-- Attempted access to a portion of the buffer that does not correspond to the associated file(for example, beyond the end of the file, including the case where another process has truncated the file). -x The end x-
Friday, February 29, 2008
C Questions 6
Peter van der Linden, in his book 'Expert C programming - Deep C secrets'
Q1.
main()
{
unsigned i=-10;
printf("i=%d\n",i);
}
Ans:
i=-10
Q2.
main()
{
unsigned i=-10;
printf("i=%u\n",i);
}
Ans:
i=4294967286
Q3. How do you include assembly instructions inside a c program?
Ans:
_asm_ volatile {"nop" "\n\t"}
Why the asm word was used here?
~To tell the assembler that it is an assembly instruction.
Why the volatile word is being used here?
~To tell the compiler, not to optimize the code here.
Q4. Though the sizeof array is Zero?
struct test {
int len;
char a[0];
}
int main()
{
struct test m1;
m1.a[0] = 'a';
printf("%c\n",m1.a[0]);
printf("sizeof=%d",sizeof(m1));
}
Q5. Write a self reproducible C program.
Ans:
char*f="char*f=%c%s%c;main()
{printf(f,34,f,34,10);}%c";
main(){printf(f,34,f,34,10);}
Note: Here is a list of other such quines written using C language.
http://www.nyx.net/~gthompso/self_c.txt
Q6. Tell me the o/p:
main()
{
char i=255;
printf("i=%d\t%c\n",i,i);
}
Ans:
i= -1
Q7.
main()
{
unsigned char i=-1;
printf("i=%d\t%c\n",i,i);
}
Ans:
i=255
Q8.
1 #include
2
3 main()
4 {
5 char *pary="string";
6 char ary[]="string";
7 printf("sizeof=%d %d\n", sizeof(ary), sizeof(pary));
8 printf("strlen=%d %d\n", strlen(ary), strlen(pary));
9 }
Ans:
sizeof=7 4
strlen=6 6
Note: machine details:
SunOS devtest6 5.8 Generic_117350-39 sun4u sparc SUNW,Ultra-80
Q9. Compile this code:
1 #include
2
3 main()
4 {
5 char *pary="string";
6 char ary[]="string";
7 const char *a="mystring";
8 char const *b="mystring";
9 // const *char c="mystring";
10 char *const d="mystring";
11 const char *const dd="mystring";
12
13 const char e[]="mystring";
14 char const f[]="mystring";
15
16 printf("sizeof=%d %d\n", sizeof(ary), sizeof(pary));
17 printf("strlen=%d %d\n", strlen(ary), strlen(pary));
18
19 a="new";
20 //a[0]='n';
21
22 b="new";
23 //b[0]='n';
24
25 d="new";
26 //d[0]='n';
27
28 dd="new";
29 //dd[0]='n';
30
31 strcpy(e,"new");
32 e[0]='0';
33
34 strcpy(f,"new");
35 f[0]='0';
36
37 }
38
Ans::
In Solaris:
devtest6:/home/jkongkon/sea>!gc
gcc -v
Reading specs from /export/tools/SunOS5.8/gcc-2.95.3/lib/gcc-lib/sparc-sun-solaris2.8/2.95.3/specs
gcc version 2.95.3 20010315 (release)
devtest6:/home/jkongkon/sea>gcc -g 2.c
2.c: In function `main':
2.c:25: warning: assignment of read-only variable `d'
2.c:28: warning: assignment of read-only variable `dd'
2.c:31: warning: passing arg 1 of `strcpy' discards qualifiers from pointer target type
2.c:32: warning: assignment of read-only location
2.c:34: warning: passing arg 1 of `strcpy' discards qualifiers from pointer target type
2.c:35: warning: assignment of read-only location
devtest6:/home/jkongkon/sea>
In Linux:
devtest21.hursley.ibm.com:/home/jkongkon/sea>gcc -v
Reading specs from /usr/lib/gcc/x86_64-redhat-linux/3.4.4/specs
Configured with: ../configure --prefix=/usr --mandir=/usr/share/man --infodir=/usr/share/info --enable-shared --enable-threads=posix --disable-checking --with-system-zlib --enable-__cxa_atexit --disable-libunwind-exceptions --enable-java-awt=gtk --host=x86_64-redhat-linux
Thread model: posix
gcc version 3.4.4 20050721 (Red Hat 3.4.4-2)
devtest21.hursley.ibm.com:/home/jkongkon/sea>cc
cc: no input files
devtest21.hursley.ibm.com:/home/jkongkon/sea>gcc -g 2.c
2.c: In function `main':
2.c:25: error: assignment of read-only variable `d'
2.c:28: error: assignment of read-only variable `dd'
2.c:31: warning: passing arg 1 of `strcpy' discards qualifiers from pointer target type
2.c:32: error: assignment of read-only location
2.c:34: warning: passing arg 1 of `strcpy' discards qualifiers from pointer target type
2.c:35: error: assignment of read-only location
devtest21.hursley.ibm.com:/home/jkongkon/sea>
Q10. What the following code will do:
void (*fp)[3] () = { do_something, do_another_thing, do_something_else };
int ch = getchar();
if (ch >= 'a' && ch <= 'c') (*(fp + ch - 'a'))(); else do_default(); Ans: The above code looks complicated, but the meaning is very simple. if((ch = getchar()) == 'a') do_something(); else if(ch = = 'b') do_another_thing(); else if(ch = = 'c') do_something_else(); else do_default(); Or ch= getchar(); switch(ch) { case 'a': { do_something(); break; } case 'b': { do_another_thing(); break; } case 'c': { do_something_else(); break; } default: do_default(); Q12:What is the side effect? malloc(); free(); free(); Q13:What is the side effect? fopen(); fclose(); fclose();
Wednesday, February 27, 2008
C Questions 5
Let's start today with something else. The English calendar of September 1752 contained only 19 days, and is the shortest month. Surprised. To know more please read this:
In September 1752 the Julian calendar was replaced with the Gregorian calendar in Great Britain and its American colonies. The Julian calendar was 11 days behind the Gregorian calendar, so 14 September got to follow 2 September on the day of the change. The result was that between 3 and 13 September, absolutely nothing happened!
To know more:
http://www.didyouknow.cd/calendar.htm
Q0. Do you know what happens when you type:
$cal 9 1752
September 1752
S M Tu W Th F S
1 2 14 15 16
17 18 19 20 21 22 23
24 25 26 27 28 29 30
And in this calendar days from 3rd till 13th are missing.
Q1. void *p++;
void *q; q++;
What p,q will point to?
Q2. DMA uses Logical address, or physical address?
Q3. What printf returns?
Q4. While in bottom half, what is the context, is it still the process context?
Q5. (MS) You are trying to use notepad, then while clicking on save, the application hangs. How will you go about debugging this? The complete source code of NOTEPAD is available with you. List down the scenarios and steps.
Q6. Tell me the o/p.
main(){
const int i=5;
i++;
printf("%d\n", i);
}
Ans:
Compilation:
warning: initialization discards qualifiers from pointer target type
warning: increment of read-only variable `i'
Result: i= 6
Q7. Tell me the o/p.
main() {
const int i =5;
int *p=&i;
p++;
printf("*p=%d", *p);
}
Ans:
Compilation:
warning message: warning: initialization discards qualifiers from pointer target type
Result:
*p=garbage value
Q8. Tell me the o/p.
main() {
const int i =5;
int *p=&i;
*p++;
printf("i=%d\n", i);
printf("*p=%d\n", *p);
}
Ans:
Compilation:
warning message: warning: initialization discards qualifiers from pointer target type
Result:
i=5
*p=garbage value
Q9. Tell me the o/p.
main() {
const int i =5;
int *p=&i;
(*p)++;
printf("i=%d\n", i);
printf("*p=%d\n", *p);
}
Ans:
Compilation:
warning message: warning: initialization discards qualifiers from pointer target type
Result:
i=6
*p=6
Q10.
main()
{
const i=9;
const int *p;
p=&i;
printf("*p=%d\n",*p);
p++;
printf("*p=%d\n",*p);
}
Ans:
*p=9
*p=garbage value
Q11. What are the differences in the following declarations:
a)
const i;
const int i;
b)
const *p;
const int *p;
int * const p;
const *int p;
int const *p;
Ans:
const int * p;
declares that p
is variable pointer to a constant integer andint const * p;
is an alternative syntax which does the same, whereas int * const q;
declares that q
is constant pointer to a variable integer and int const * const p1;
declares that p1
is constant pointer to a constant integer. Basically ‘const’ applies to whatever is on its immediate left (other than if there is nothing there in which case it applies to whatever is its immediate right).Q12.
int func()
{
printf("Hi");
func();
}
int main(){ func();}
What will be the o/p?
Ans:
Stack overflow, why?
The stack frame for func needs to store the entry and exit point each time func() is recursively called. This will fill up the stack, and hence overflow.
The func() is calling printf(), and in the printf's stack frame you will put the string argument "Hi", and not in the stack frame of func().
Q13.
main()
{
const int i=10;
i=20;
printf(i=%d\n",i);
}
Ans:
warning: assignment of read-only variable `i'
Result:
i=20
Now, tell me where the const variable is stored?
Subscribe to:
Posts (Atom)