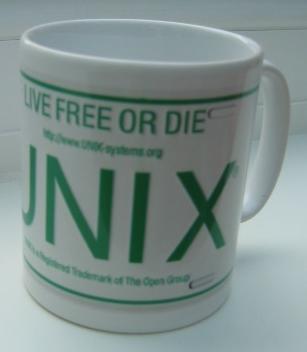
Hello World!
1.
main()
{
printf("Hello World!\n");
}
>gcc 1.c
> ./a.out
Hello World!
Here is a space where I put some of my C questions collection.
2.
Here is a way to print/execute both if part and else part in an if-then-else construct.
main()
{
if(!printf("Hello ")){}
else
printf("World!");
}
>gcc 2.c
> ./a.out
Hello World!
3.
Sometime serious about how malloc works. Even if you pass the address of a member (variable) while you malloc a struct, see how free works. It can free up the space using that address also.
main()
{
struct a {
int b;
int c;
int d;
};
struct a *ptr;
ptr = (struct a *) malloc(sizeof(struct a ));
free(ptr->c);
}
4.
Here is something about address alignment. See how memory addresses are aligned in case of a struct.
main()
{
struct i{
int a; //4
double b; //8
char * c; //4
char d[7]; //8
short e; //4
int f; //4
};
printf("sizeof(i)= %d", sizeof(struct i));
}
Ans: 4+8+4+8+4+4=32
5.
Inside into the printf function.
# include < stdio.h >
void main()
{
printf(NULL) ;
return ;
}
> ./a.out
>
6.
# include < stdio.h >
{
int i = 0;
printf(i) ;
return ;
}
Ans:
>
# include < stdio.h >
{
int i = 0;
printf("%f\n", i) ;
return ;
}
# include < stdio.h >
{
int i = 0;
printf("%f %d\n", i) ;
return ;
}
#include <stdio.h>
{
printf("%s",NULL) ;
return ;
}
> ./a.out
(null)
void main()
{
int i = -10;
printf("%s\n",i) ;
return ;
}
will result in "Segmentation fault" if the value of i is other than zero.
8.
This will work.
main()
{
char *a;
char b[13]="hello world";
strcpy(a,b);
printf("%s", a);
}
9.
main()
{
printf("%%",7);
}
10.
Array boundary condition behavior
main() {
int a[4]={1,2,3,4};
printf("%u %u", &a, &a+1);
}
>gcc 10.c
> ./a.out
3220829536 3220829552
11.
Again array boundary behaviour.
main() {
int a[3]={1,2,3,4};
printf("%u %u", &a, &a+1);
}
gcc 11.c
> gcc 6.c
11.c: In function `main':
11.c:2: warning: excess elements in array initializer
11.c:2: warning: (near initialization for `a')
> ./a.out
3220516784 3220516796
12.
main()
{
int i=10;
i=i++ + ++i;
printf("i=%d",i);
}
>./a.out
i=23
13. To find greatest among two numbers without using relational operator.
int main()
{
int a,b,c,d;
printf("Enter any two nos for a and b");
scanf("%d%d",&a,&b);
c=a-b;
d=b-a;
while(c && d)
{
--c;
--d;
}
if(c)
printf("b is greatest");
else
printf("a is greatest");
}
Try this...