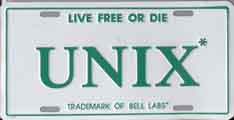
1.
See how it behaves when you have less space than required, here it is the string-copy operation in target.
main()
{
char *a;
//a> char a[];
//b> char a[12];
//c> char a[10];
char b[10]="Hello World"; //sizeof=11
strcpy(a,b);
printf("%s\n", a);
}
[guest@caramel mine]$ ./a.out
Segmentation Fault
[guest@caramel mine]$
a> Segmentation Fault
b> Hello World
c> Segmentation Fault
2.
Big Blue or Not?
Big Blue:
main()
{
int i=1;
char *ch = &i; // here ch points to least significant byte.
if(*ch == 1) // check least significant byte has value ?
{
printf("Littel Endian");
}
else
printf ("Big Endian");
}
[kongkon@~ >]gcc 2.c
2.c: In function `main':
2.c:5: warning: initialization from incompatible pointer type
[kongkon@~ >]./a.out
Big Endian[kongkon@~ >]
[kongkon@~ >]uname -a
Linux ltsbuild.austin.ibm.com 2.6.5-7.97-pseries64 #1 SMP Fri Jul 2 14:21:59 UTC 2004 ppc64 ppc64 ppc64 GNU/Linux
[kongkon@~ >]
Not:(intel:Leap Ahead)
[guest@caramel jnk]$ gcc 2.c
2.c: In function `main':
2.c:5: warning: initialization from incompatible pointer type
[guest@caramel jnk]$ ./a.out
Littel Endian[guest@caramel jnk]$ uname -a
Linux caramel.in.ibm.com 2.4.22-1.2199.nptl #1 Wed Aug 4 12:21:48 EDT 2004 i686 i686 i386 GNU/Linux
3.
Writing printf statement without using semicolon at the end:
#include
main()
{
if( ! printf("Write watever in this") )
{
// you will never reach here
}
while (! Printf("Here too u can write ") )
{
// You will never reach here
}
}
4.
Finding a number is a power of 2 in single line program in c:
main()
{
int n;
( n & n-1 ) ? printf("Number is power of 2") : printf("Number is power of 2") ;
}
5. extern var
main()
{
extern int i;
i=20;
printf("%d",i);
}
kongkon@ltsbml:~> gcc 5.c
/tmp/cckHzObg.o(.text+0x16): In function `main':: undefined reference to `i'
/tmp/cckHzObg.o(.text+0x1e): In function `main':: undefined reference to `i'
/tmp/cckHzObg.o(.text+0x22): In function `main':: undefined reference to `i'
/tmp/cckHzObg.o(.text+0x2e): In function `main':: undefined reference to `i'
collect2: ld returned 1 exit status
kongkon@ltsbml:~> gcc -c 5.c
kongkon@ltsbml:~>
There is a linker error, but no error at the time of compilation.
6. How const works?
main()
{
const int x=get();
printf("%d\n",x);
}
get(){return(20);}
kongkon@ltsbml:~> gcc 6.c
kongkon@ltsbml:~> ./a.out
20
kongkon@ltsbml:~>gcc --version
gcc (GCC) 3.3.3 (SuSE Linux)Copyright (C) 2003 Free Software Foundation, Inc.
This is free software; see the source for copying conditions. There is NOwarranty; not even for MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE.
7. Writing C program without main()
In turbo C, use this
#pragma startup my_main
my_main(){
...
...
}
8. Executing C programming without using ; to end the line.
Method A:
int main()
{
if (!printf("Hello"))
{}
}
Method B:
#define ABC ;
printf("abc")ABC
9. At the ground ZERO!!
kongkon@ltsbml:~> cat 9.c
main()
{
int a[0];
printf("sizeof a = %d\n", sizeof(a));
}
kongkon@ltsbml:~> gcc 9.c
kongkon@ltsbml:~> ./a.out
sizeof a = 0
kongkon@ltsbml:~>
10.
It's a matter of recreatibility. This is self reproducing code.
kongkon@ltsbml:~> cat 10.c
char *s="char *s=%c%s%c;main() { printf(s,34,s,34); }"; main() { printf(s,34,s,34); }
kongkon@ltsbml:~> gcc 10.c
kongkon@ltsbml:~> a.out
char *s="char *s=%c%s%c;main() { printf(s,34,s,34); }";main() { printf(s,34,s,34); }kongkon@ltsbml:~>
11. void or no-void
main()
{
add(0, 13); //pass any number of arguments, it will work, NO Warnings!
}
int add(void)
{
printf("Hello World");
}
12. Stack goes downward/upward?
To check whether the C program stack is growing upwards, or downwards the following C code will help you out.
int main()
{
int i,j;
printf("%u %u",&i,&j);
}
13.Size does matters!
#define SIZE(any_var) ((char *)((&any_var) +1) - (char *) (&any_var))
This will give you sizeof any variable...
Thanks a lot for reading my blog.